We can use Eclipse's External Tools capabilities to perform a mavenSW "archetype:create" goal from EclipseSW. We can start by opening the Eclipse External Tools Dialog.

I created an external tool configuration with the following settings:
Name: | mvn archetype~create |
Location: | C:\dev\apache-maven-2.0.8\bin\mvn.bat |
Working Directory: | ${workspace_loc} |
Arguments: | archetype:create -DgroupId=${string_prompt:groupId} -DartifactId=${string_prompt:artifactId} |
I used a ~ instead of a : for the configuration Name since Eclipse doesn't allow colons as part of a configuration name. I set mvn.bat (maven) as the Location (tool) to execute. I set the Working Directory to be the Eclipse variable ${workspace_loc}, which represents the Eclipse workspace folder. For the Arguments, I specified to perform the "archetype:create" goal. The string_prompt variables specify that the user will be prompted to enter the groupId and artifactId values when the external configuration is run.
The External Tools Dialog is shown below.

If we run the new "mvn archetype~create" external tool configuration, we get prompted for the groupId value. I entered "com.maventest" and clicked OK.
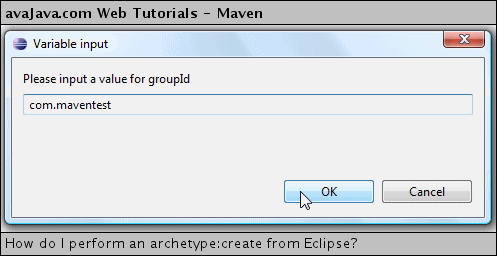
Next, we get prompted for the artifactId value. I entered "mytest" and clicked OK.

In the Eclipse Console view, we see that the archetype:create goal is executed.
Console Output
[INFO] Scanning for projects... [INFO] Searching repository for plugin with prefix: 'archetype'. [INFO] ------------------------------------------------------------------------ [INFO] Building Maven Default Project [INFO] task-segment: [archetype:create] (aggregator-style) [INFO] ------------------------------------------------------------------------ [INFO] Setting property: classpath.resource.loader.class => 'org.codehaus.plexus.velocity.ContextClassLoaderResourceLoader'. [INFO] Setting property: velocimacro.messages.on => 'false'. [INFO] Setting property: resource.loader => 'classpath'. [INFO] Setting property: resource.manager.logwhenfound => 'false'. [INFO] ************************************************************** [INFO] Starting Jakarta Velocity v1.4 [INFO] RuntimeInstance initializing. [INFO] Default Properties File: org\apache\velocity\runtime\defaults\velocity.properties [INFO] Default ResourceManager initializing. (class org.apache.velocity.runtime.resource.ResourceManagerImpl) [INFO] Resource Loader Instantiated: org.codehaus.plexus.velocity.ContextClassLoaderResourceLoader [INFO] ClasspathResourceLoader : initialization starting. [INFO] ClasspathResourceLoader : initialization complete. [INFO] ResourceCache : initialized. (class org.apache.velocity.runtime.resource.ResourceCacheImpl) [INFO] Default ResourceManager initialization complete. [INFO] Loaded System Directive: org.apache.velocity.runtime.directive.Literal [INFO] Loaded System Directive: org.apache.velocity.runtime.directive.Macro [INFO] Loaded System Directive: org.apache.velocity.runtime.directive.Parse [INFO] Loaded System Directive: org.apache.velocity.runtime.directive.Include [INFO] Loaded System Directive: org.apache.velocity.runtime.directive.Foreach [INFO] Created: 20 parsers. [INFO] Velocimacro : initialization starting. [INFO] Velocimacro : adding VMs from VM library template : VM_global_library.vm [ERROR] ResourceManager : unable to find resource 'VM_global_library.vm' in any resource loader. [INFO] Velocimacro : error using VM library template VM_global_library.vm : org.apache.velocity.exception.ResourceNotFoundException: Unable to find resource 'VM_global_library.vm' [INFO] Velocimacro : VM library template macro registration complete. [INFO] Velocimacro : allowInline = true : VMs can be defined inline in templates [INFO] Velocimacro : allowInlineToOverride = false : VMs defined inline may NOT replace previous VM definitions [INFO] Velocimacro : allowInlineLocal = false : VMs defined inline will be global in scope if allowed. [INFO] Velocimacro : initialization complete. [INFO] Velocity successfully started. [INFO] [archetype:create] [INFO] Defaulting package to group ID: com.maventest [INFO] ---------------------------------------------------------------------------- [INFO] Using following parameters for creating Archetype: maven-archetype-quickstart:RELEASE [INFO] ---------------------------------------------------------------------------- [INFO] Parameter: groupId, Value: com.maventest [INFO] Parameter: packageName, Value: com.maventest [INFO] Parameter: package, Value: com.maventest [INFO] Parameter: artifactId, Value: mytest [INFO] Parameter: basedir, Value: C:\dev\workspace [INFO] Parameter: version, Value: 1.0-SNAPSHOT [INFO] ********************* End of debug info from resources from generated POM *********************** [INFO] Archetype created in dir: C:\dev\workspace\mytest [INFO] ------------------------------------------------------------------------ [INFO] BUILD SUCCESSFUL [INFO] ------------------------------------------------------------------------ [INFO] Total time: 1 second [INFO] Finished at: Thu Jan 31 13:35:05 PST 2008 [INFO] Final Memory: 5M/9M [INFO] ------------------------------------------------------------------------
If we examine the file system, we can see that the "mytest" project was created in the Eclipse workspace. On my machine this was at C:\dev\workspace\mytest
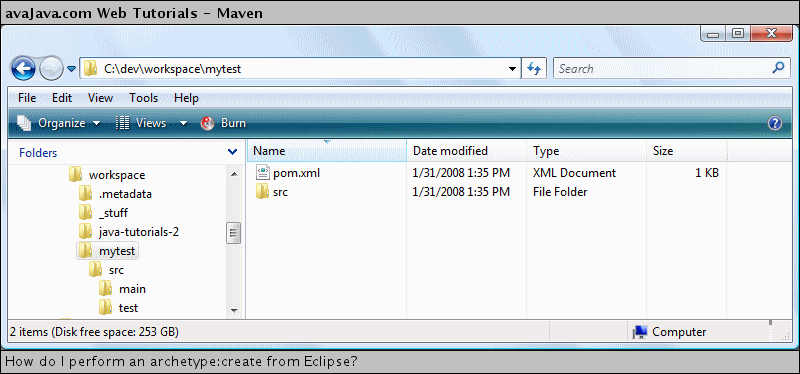
At this point, we have created the project and we can see it in the file system, but it is not visible yet in Eclipse. In another tutorial, we'll examine how to import a maven project into Eclipse.